.width()返回值: 数字
描述: 获取匹配元素集中第一个元素的当前计算宽度。
.css( "width" )
和 .width()
之间的区别在于,后者返回一个无单位的像素值(例如,400
),而前者返回一个带有单位的完整值(例如,400px
)。当需要在数学计算中使用元素宽度时,建议使用 .width()
方法。
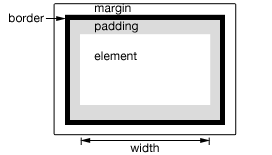
此方法还可以找到窗口和文档的宽度。
1
2
3
4
5
|
|
请注意,.width()
将始终返回内容宽度,无论 CSS box-sizing
属性的值如何。从 jQuery 1.8 开始,这可能需要检索 CSS 宽度加上 box-sizing
属性,然后在元素具有 box-sizing: border-box
时从每个元素中减去任何潜在的边框和填充。为了避免这种开销,请使用 .css( "width" )
而不是 .width()
。
注意:虽然 style
和 script
标签在绝对定位并设置 display:block
时会报告 .width()
或 height()
的值,但强烈建议不要在这些标签上调用这些方法。除了是一种不好的做法之外,结果也可能不可靠。
其他说明
-
由尺寸相关 API(包括
.width()
)返回的数字在某些情况下可能是小数。代码不应假设它是一个整数。此外,当用户缩放页面时,尺寸可能不正确;浏览器不提供 API 来检测这种情况。 -
当元素或其父元素隐藏时,
.width()
报告的值不能保证准确。要获得准确的值,请确保元素在使用.width()
之前可见。jQuery 将尝试暂时显示然后重新隐藏元素以测量其尺寸,但这不可靠,而且(即使准确)也会严重影响页面性能。此显示和重新隐藏测量功能可能会在 jQuery 的未来版本中删除。
示例
显示各种宽度。请注意,这些值来自 iframe,因此可能比您预期的要小。黄色突出显示显示 iframe 主体。
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
|
|
演示
.width( value )返回值:jQuery
描述:设置匹配元素集中每个元素的 CSS 宽度。
-
添加版本:1.0.width( value )
-
value表示像素数的整数,或一个整数加上一个可选的度量单位(作为字符串)附加。
-
-
添加版本:1.4.1.width( function )
-
function返回要设置的宽度的函数。接收集合中元素的索引位置和旧宽度作为参数。在函数中,
this
指的是集合中的当前元素。
-
当调用 .width("value")
时,value 可以是字符串(数字和单位)或数字。如果仅为 value 提供数字,jQuery 假设为像素单位。但是,如果提供字符串,则可以使用任何有效的 CSS 测量值作为宽度(例如 100px
、50%
或 auto
)。请注意,在现代浏览器中,CSS 宽度属性不包括填充、边框或边距,除非使用 box-sizing
CSS 属性。
如果未指定显式单位(如“em”或“%”),则假定为“px”。
请注意,.width("value")
设置框的内容宽度,而与 CSS box-sizing
属性的值无关。
示例
第一次点击每个 div 时,更改其宽度(并更改其颜色)。
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
|
|